Reading the failures
Let's take this Username
modddel again as an example :
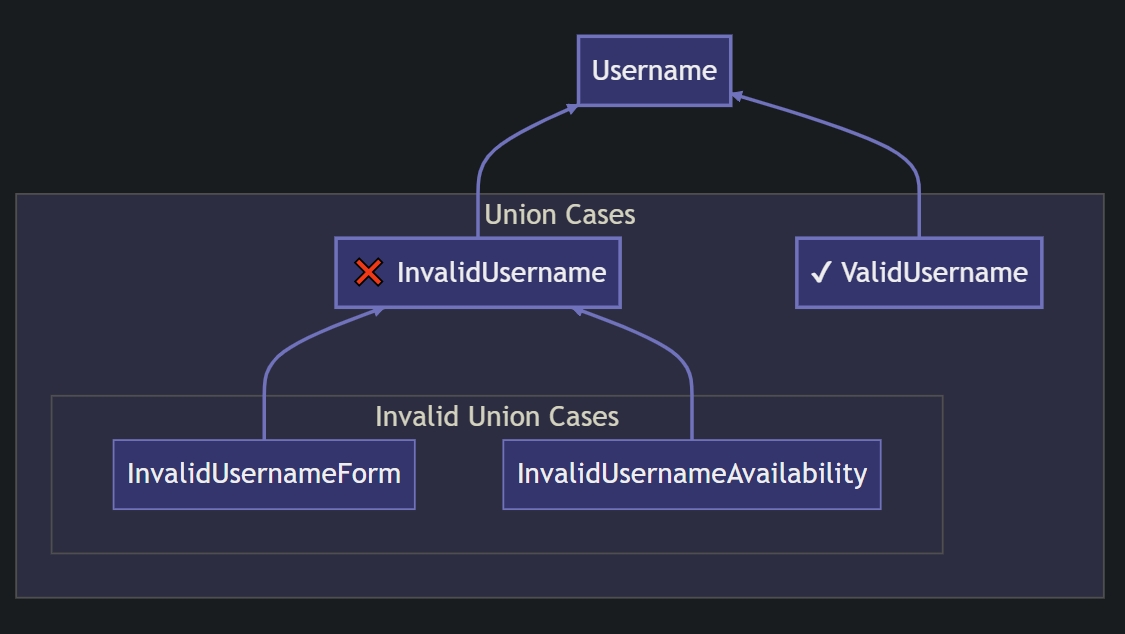
The 'form' validationStep contains two validations : 'length' validation and 'characters' validation. The 'availability' validationStep contains only one validation : 'reserved' validation.
You can easily access the failure(s) of an invalid-step union-case :
username.mapOrNull(
invalidAvailability: (invalidUsernameAvailability) {
/// InvalidUsernameAvailability has only one validation, and thus only one
/// failure that it can hold.
///
/// For this reason, the failure is not nullable.
///
UsernameReservedFailure failure = invalidUsernameAvailability.reservedFailure;
},
invalidForm: (invalidUsernameForm) {
/// InvalidUsernameForm has two validations, and thus can hold one or two
/// failures.
///
/// For this reason, the failures are nullable.
///
UsernameCharactersFailure? failure1 = invalidUsernameForm.charactersFailure;
UsernameLengthFailure? failure2 = invalidUsernameForm.lengthFailure;
/// You can use the "hasFailure" getters to check if the invalid-step
/// union-case has a particular failure.
///
if (invalidUsernameForm.hasCharactersFailure) {
// At this point, the charactersFailure is not null.
final failure = invalidUsernameForm.charactersFailure!;
}
if (invalidUsernameForm.hasLengthFailure) {
// At this point, the lengthFailure is not null.
final failure = invalidUsernameForm.lengthFailure!;
}
},
);
You can also access a list of all the failures of an invalid union-case, using the failures
getter :
username.mapValidity(
valid: (validUsername) {},
invalid: (invalidUsername) {
/// We can access the list of failures from the abstract invalid union-case
///
final failures = invalidUsername.failures;
/// We can also access it from each invalid-step union-case
///
invalidUsername.mapOrNull(
invalidForm: (invalidUsernameForm) {
final failures = invalidUsernameForm.failures;
},
);
},
);